A Beginner’s Guide to CRUD Operations in DynamoDB
Objective
The objective of this article is to help you understand CRUD Operations in DynamoDB. In this article, you will learn about to create a DynamoDB table using the CloudFormation template and perform the create, read, update, and delete data using the AWS CLI tool.
Prerequisites
- An AWS account with permissions to access DynamoDB
- Basic knowledge of NoSQL database – DynamoDB
Step-1: Create a DynamoDB table using CloudFormation
Create a new file called “create-dynamo-db-cf.template” and paste the following code to create a DynamoDB Table.
{
"AWSTemplateFormatVersion": "2010-09-09",
"Parameters": {
"TableName": {
"Type": "String",
"Default" : "CustomerTable",
"Description": "Name of the DynamoDB table to be created"
},
"ReadCapacity": {
"Type": "Number",
"Default" : 5,
"Description": "The maximum number of strongly consistent reads per second"
},
"WriteCapacity": {
"Type": "Number",
"Default" : 5,
"Description": "The maximum number of writes per second"
},
"CapacityMode": {
"Type": "String",
"Description": "The capacity mode to use for the DynamoDB table",
"AllowedValues": [
"PAY_PER_REQUEST",
"PROVISIONED"
]
}
},
"Resources": {
"DynamoDBTable": {
"Type": "AWS::DynamoDB::Table",
"Properties": {
"AttributeDefinitions": [
{
"AttributeName": "Id",
"AttributeType": "N"
}
],
"KeySchema": [
{
"AttributeName": "Id",
"KeyType": "HASH"
}
],
"BillingMode": {"Ref" : "CapacityMode"},
"ProvisionedThroughput": {
"ReadCapacityUnits": {"Ref" : "ReadCapacity"},
"WriteCapacityUnits": {"Ref" : "WriteCapacity" }
},
"TableName": {"Ref" : "TableName"}
}
}
},
"Outputs": {
"TableName": {
"Description": "Name of the DynamoDB table",
"Value": {
"Ref": "DynamoDBTable"
}
}
}
}
This cloud formation template is used to create a DynamoDB table with the following parameters:
- AWSTemplateFormatVersion: specifies the format version of the template
- Parameters: declares input parameters that can be passed into the template when it is run
- TableName: specifies the name of the DynamoDB table to be created
- ReadCapacity: specifies the maximum number of strongly consistent reads per second for the DynamoDB table
- WriteCapacity: specifies the maximum number of writes per second for the DynamoDB table
- CapacityMode: specifies the capacity mode to use for the DynamoDB table, with the allowed values of PAY_PER_REQUEST or PROVISIONED
- Resources: declares the resources that are created by the template, in this case, a DynamoDB table with the specified properties
- DynamoDBTable: specifies the type of resource to create – AWS::DynamoDB::Table
- AttributeDefinitions: specifies the attributes that define the key schema of the DynamoDB table
- KeySchema: specifies the key attributes of the DynamoDB table
- BillingMode: specifies the billing mode for the DynamoDB table using the value of the CapacityMode parameter
- ProvisionedThroughput: specifies the provisioned throughput for the DynamoDB table using the values of the ReadCapacity and WriteCapacity parameters
- Outputs: declares the output values that can be returned by the template when it is run.
Step-2: Deploy cloud formation template from AWS console
- Login to your AWS Console and navigate to the “CloudFormation” service
- Click on the “Create stack” button to start the cloud formation creation process.
- Upload your created template file “create-dynamo-db-cf.template”, Click on “Next”
- Specify the stack details, Click on “Next”, “NEXT” and “Submit”. Wait until stack creation is complete.
- Using AWS Console search, navigate to the “DynamoDB” service. Verify that the DynamoDB table gets created by the cloud formation template.
Step-3: Create, Read, Update, and Delete operations using AWS CLI command
Here are example AWS CLI commands for CRUD operations on a DynamoDB table named “CustomerTable” with a primary key “Id” for the model
Customer
{
"Id": int,
"Name": "string",
"Email": "string"
"Address": "string",
}
Following commands output based on Windows environment and AWS CLI2.
Create a new item – A new customer entry in DynamoDB table
aws dynamodb put-item --table-name CustomerTable --item "{\"Id\":{\"N\": \"123\"},\"Name\":{\"S\":\"John Wick\"},\"Email\":{\"S\": \"johnwick@example.com\"}, \"Address\": {\"S\": \"123 Main St\"}}"
Read item: Get the customer details with an Id of 123
aws dynamodb get-item --table-name CustomerTable --key "{ \"Id\": {\"N\": \"123\"} }"
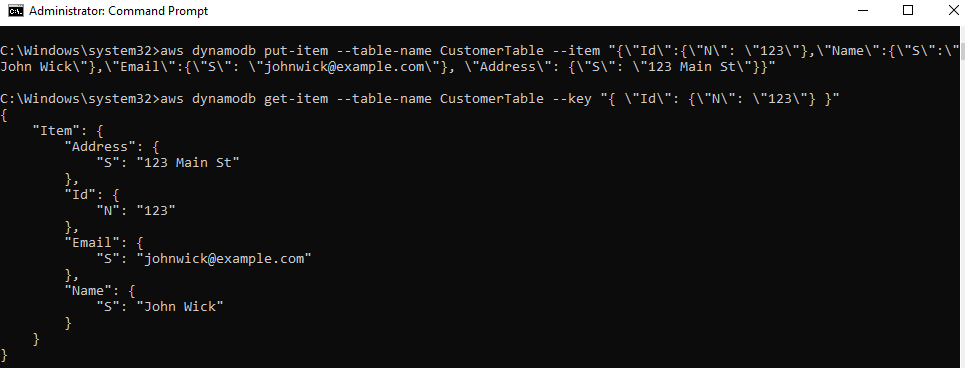
Update item: update existing customer details with Id of 123
aws dynamodb update-item --table-name CustomerTable --key "{\"Id\": {\"N\": \"123\"}}" --update-expression "SET #name = :name, #email = :email, #address = :address" --expression-attribute-names "{\"#name\": \"Name\", \"#email\": \"Email\", \"#address\": \"Address\"}" --expression-attribute-values "{\":name\": {\"S\": \"James\"}, \":email\": {\"S\": \"James@example.com\"}, \":address\": {\"S\": \"456 Main St\"}}"
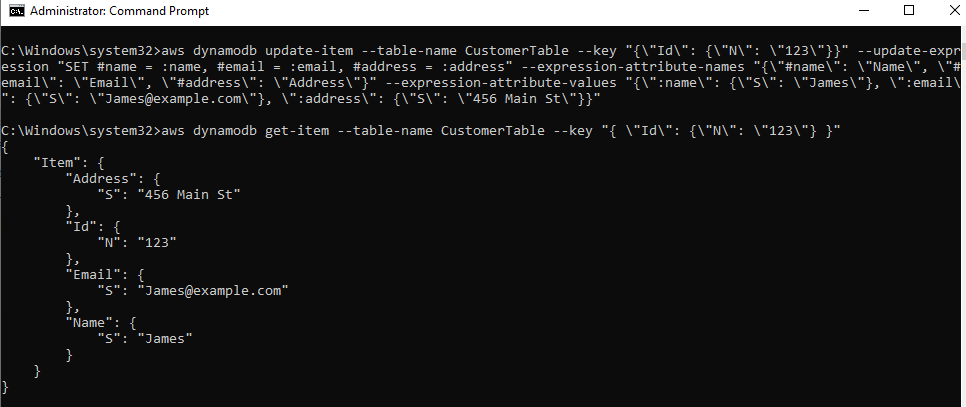
Delete item: Delete customer details from table with Id of 123
aws dynamodb delete-item --table-name CustomerTable --key "{ \"Id\": {\"N\": \"123\"} }"

Note: Delete the cloud formation stack “create-dynamo-db-cf.template” to delete DynamoDB.